Organizing Variables and Function
Before we start to discuss about structures and class. Let us create a set of variables and function that allow us to use in an contact management app.
Creating Contact List App
Our contact list will contain the following:
First name, Last name, Mobile number, Email and Twitter handle
We also need to create an array that hold the contact list. First we need a function to display the contact list. In addition, we will need a function to load data. In our example, we will create new contact list instead of loading from database.
Similarly, we need a function to store store data.
Besides, we will need a set to function to add contact, display contact, remove contact.
Our data requirement is as follows:
- Array for contact list
- First name
- Last name
- Mobile number
- Email
- Twitter handle
We will create a tuple that contain name, mobile, email and twitter handle.
List of function is as follows:
- displayContactList
- loadContactList
- storeContactList
- addContact
- removeContact
- editContact
For the contact list, we create an array of tuple as shown below:
// Variables
var contactList = [(firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)]() |
Listed below are the various function:
loadContactList: For reduce complexity, we will load static data
func loadContactList () {
let contact1 = (firstName: "John", lastName: "Smith", mobileNumber:82736487, email:"john.smith@outlook.com",twitterHandle:"@johmsmith") let contact2 = (firstName: "Steve", lastName: "Carpenter", mobileNumber:92873432, email:"steve.carpenter@outlook.com",twitterHandle:"@stevecarp") let contact3 = (firstName: "Mathew", lastName: "Emerson", mobileNumber:9876547, email:"mathew.emerson@gmail.com",twitterHandle:"@matemerson") contactList += [contact1, contact2, contact3] print("Load complete") } |
displayContactList: This function just display everything in the array and also print the total
func displayContactList (myContactList:[(firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)]) {
let total = myContactList.count
for (index, eachContact) in myContactList.enumerated() {
print("Contact \(index)")
print("First Name: \(eachContact.firstName) Last Name: \(eachContact.lastName)")
print("Mobile: \(eachContact.mobileNumber)")
print("Email: \(eachContact.email)")
print("Twitter: \(eachContact.twitterHandle)")
}
print("total count = \(total)")
print("-----------------------------------------")
}
|
storeContactList: We will not store anything. Instead we will just display the list to confirm what will be stored
func storeContactList () {
print("Contact list stored!")
displayContactList(myContactList: contactList)
}
|
addContact: We will add contact by passing the contact info to the function
func addContact (myContact: (firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)) {
contactList.append(myContact)
print("contact added!")
}
|
editContact: For editing, we will need to pass the index number of the contact we want to change and at the same time passed the amended contact to the function.
func editContact (index: Int, myContact: (firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)) {
contactList[index] = myContact } |
removeContact: For removing contact, we just need the index of the contact to be removed.
func removeContact (index: Int) {
contactList.remove(at: index) print("contact removed") } |
Please note that this example was created to illustrate the need for organizing our data and functions. It is greatly simplified just for illustration purpose.
To test the working of the code, we have a list of instruction that will test and confirm each function.
The following are list of instruction to execute in sequential order
loadContactList()
displayContactList(myContactList: contactList) addContact(myContact: (firstName: "Tom", lastName: "Hilton", mobileNumber: 19289283, email: "tom.hilton@gmail.com", twitterHandle: "@tomh")) displayContactList(myContactList: contactList) removeContact(index: 1) displayContactList(myContactList: contactList) editContact(index: 2, myContact: (firstName: "Eric", lastName: "Emerson", mobileNumber: 9876547, email: "eric.emerson@gmail.com", twitterHandle: "@eric")) displayContactList(myContactList: contactList) storeContactList() |
The following screenshot are the result for each instruction:
Load Data
Display Data
Add Contact: The result shows 4 contact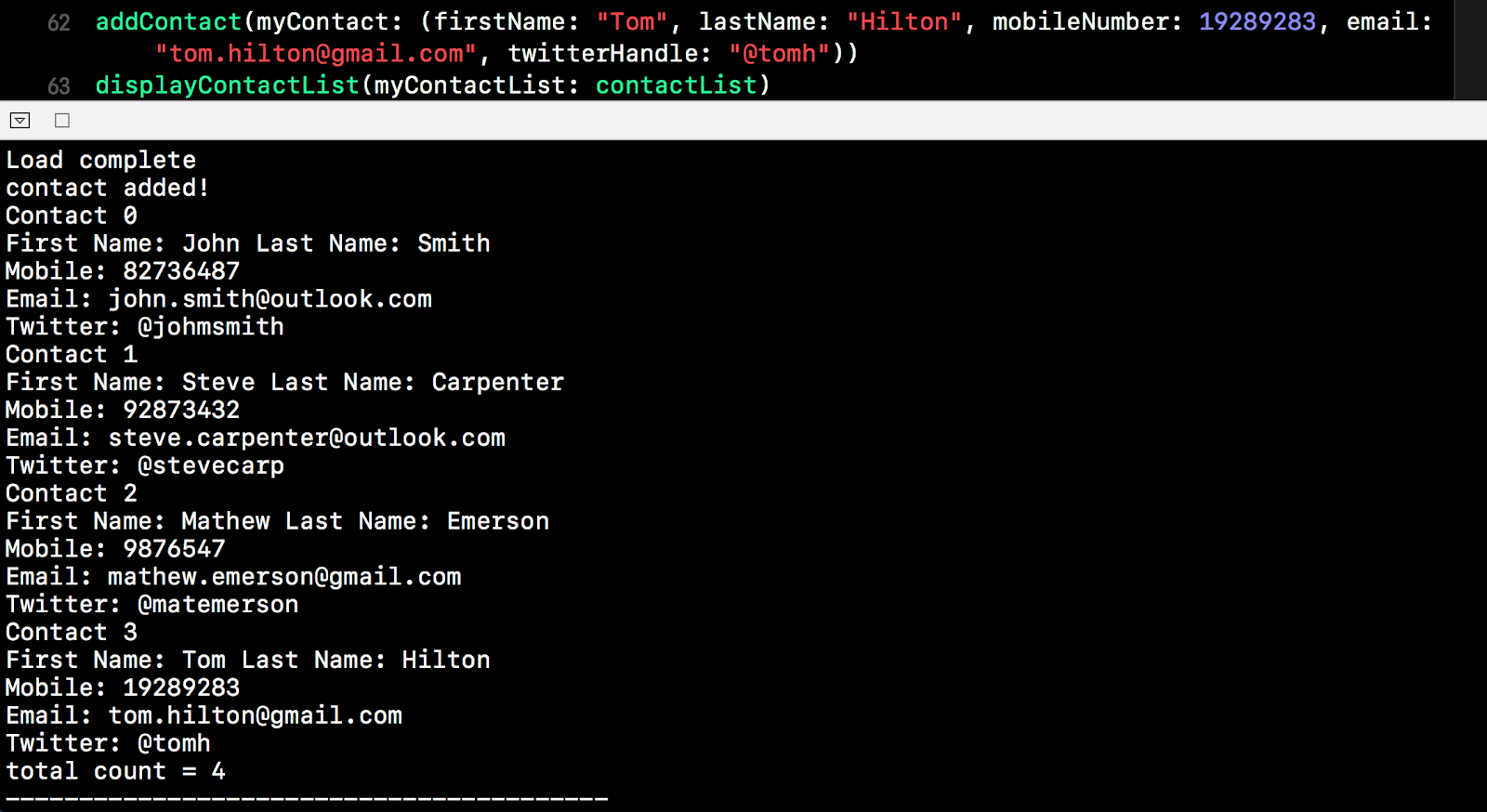
Remove Contact: After removing 2nd contact, total contact goes back to 3
Edit Contact: Please look out for changes, we change mathew emerson to eric emerson
Store Contact: Instead of storing, we will be listing the contacts that will be stored.
The entire code is listed here:
import UIKit
// Variables var contactList = [(firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)]() // Function func loadContactList () { let contact1 = (firstName: "John", lastName: "Smith", mobileNumber:82736487, email:"john.smith@outlook.com",twitterHandle:"@johmsmith") let contact2 = (firstName: "Steve", lastName: "Carpenter", mobileNumber:92873432, email:"steve.carpenter@outlook.com",twitterHandle:"@stevecarp") let contact3 = (firstName: "Mathew", lastName: "Emerson", mobileNumber:9876547, email:"mathew.emerson@gmail.com",twitterHandle:"@matemerson") contactList += [contact1, contact2, contact3] print("Load complete") } func displayContactList (myContactList:[(firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)]) { let total = myContactList.count for (index, eachContact) in myContactList.enumerated() { print("Contact \(index)") print("First Name: \(eachContact.firstName) Last Name: \(eachContact.lastName)") print("Mobile: \(eachContact.mobileNumber)") print("Email: \(eachContact.email)") print("Twitter: \(eachContact.twitterHandle)") } print("total count = \(total)") print("-----------------------------------------") } func storeContactList () { print("Contact list stored!") displayContactList(myContactList: contactList) } func addContact (myContact: (firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)) { contactList.append(myContact) print("contact added!") } func removeContact (index: Int) { contactList.remove(at: index) print("contact removed") } func editContact (index: Int, myContact: (firstName: String, lastName: String, mobileNumber:Int, email:String,twitterHandle:String)) { contactList[index] = myContact }
// Code execution
loadContactList() displayContactList(myContactList: contactList) addContact(myContact: (firstName: "Tom", lastName: "Hilton", mobileNumber: 19289283, email: "tom.hilton@gmail.com", twitterHandle: "@tomh")) displayContactList(myContactList: contactList) removeContact(index: 1) displayContactList(myContactList: contactList) editContact(index: 2, myContact: (firstName: "Eric", lastName: "Emerson", mobileNumber: 9876547, email: "eric.emerson@gmail.com", twitterHandle: "@eric")) displayContactList(myContactList: contactList) storeContactList() |
Complexity of Code and Organizing Code
As you can see, a very simple app contains many function and if we are to add additional features and functionality. The complexity increase. For decades software engineers have been trying to improve the programming methodology so that it is easier to to organize all the functions.
With the introduction of tuple, we can organize all the related variables for each contact. In C and Objective-C, we can only achieve such grouping using structure.
For older programming language, structure help to organized data, however, all the function will still be situated anywhere in a project. To organize the data and and related function, we introduce class or object oriented programming. This help us to maintain the code and allow us to reuse the code for other purpose.
In Swift, we can use tuple to group variable, however, tuple is best use for temporary holder and it is not recommended to store persistent data.
In Swift, structure or struct can organize data and code. We can incorporate functions/methods in a structure. This is one of the main difference from Swift's structure implementation versus older language implementation of structure. However, class in Swift offer more versatility and reusability.
We will explore the difference between structure and class in the later session.
***
No comments:
Post a Comment